SEOPress natively offers dozens of dynamic variables to use in your metas such as the title or the meta description. You can also use them in your schemas. Can’t find what you are looking for in the predefined list? Create your own!
Step 1 – register your dynamic variable
Copy and paste this code to your functions.php
of your child theme or theme:
function sp_titles_template_variables_array($array) { $array[] = '%%my-custom-global-variable%%'; return $array; } add_filter('seopress_titles_template_variables_array', 'sp_titles_template_variables_array');
At line 2, we add our new dynamic variable to the array.
Replace %%my-custom-global-variable%%
by the one you would like to use.
Eg: %%seo%%
Step 2 – display the value in source code
Now we have registered our new dynamic variable, we have to tell SEOPress which data to display in source code for search engines.
Copy and paste this code after the previous one in your functions.php
file:
function sp_titles_template_replace_array($array) { //escape your values! $array[] = esc_attr(wp_strip_all_tags('Best WordPress SEO plugin')); return $array; } add_filter('seopress_titles_template_replace_array', 'sp_titles_template_replace_array');
At line 3, we add our value to the array, eg, “Best WordPress SEO plugin
“.
You can do what you want here with PHP functions: be imaginative!
Step 3 – add your dynamic variable to the drop-down list
Standard SEO metabox
function sp_get_dynamic_variables($array){ $array['%%my-custom-global-variable%%'] = 'My new dynamic variable title'; return $array; } add_filter('seopress_get_dynamic_variables', 'sp_get_dynamic_variables');
Universal SEO metabox / custom schema
For the Universal SEO metabox and the custom schema, the code is different:
Step 4 – use our new dynamic variable in your meta
Edit a post, go to our SEO metabox and enter your new variable in title field for example:
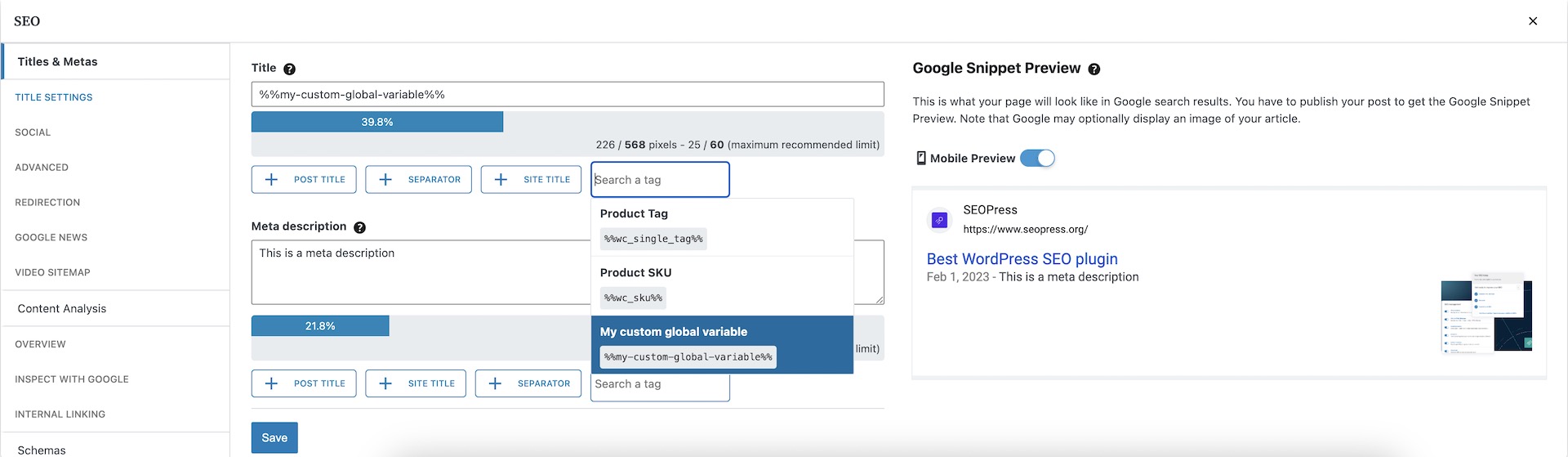
Et voilà !
How-to add several dynamic variables?
Here an example, save your post after adding the variables to the standard SEO metabox:
function sp_titles_template_variables_array($array) { $array[] = '%%my-custom-global-variable%%'; $array[] = '%%another-custom-global-variable%%'; return $array; } add_filter('seopress_titles_template_variables_array', 'sp_titles_template_variables_array'); function sp_titles_template_replace_array($array) { $array[] = esc_attr(wp_strip_all_tags( 'This is a variable' )); $array[] = esc_attr(wp_strip_all_tags( 'This is another variable' )); return $array; } add_filter('seopress_titles_template_replace_array', 'sp_titles_template_replace_array'); function sp_get_dynamic_variables($array){ $array['%%my-custom-global-variable%%'] = 'My new dynamic variable title'; $array['%%another-custom-global-variable%%'] = 'Another dynamic variable title'; return $array; } add_filter('seopress_get_dynamic_variables', 'sp_get_dynamic_variables');